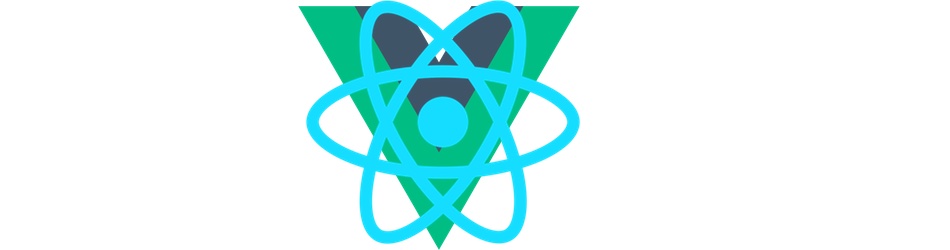
Intro
This post should serve as general observations and remarks on my own personal experience using both Vue and React for production applications and services, as opposed to simply drawing you in to a VUE VS. REACT clickbait fest.
Background of my use-cases
I began using React at the beginning of 2015. My first big use of it was on a project for Cisco, building a real-time test automation platform with websockets. Since then, I've used it on countless personal projects; Ekko and Top Spot to name a couple. My experience with Vue began a little later, this time in April 2017, on a client project for Soho House; specifically The Ned. Since then, I've built Readme App with Vue.
What follows is an informal, loose account of the differences, strengths and highlights between the two libraries.
Obligatory disclaimer: this really was my own experiences. There's inevitably multiple solutions to a problem, so my comparisons are based on my own approaches.
Stateless functional components
One of my favourite things about React: The conciseness of building a UI. Stateless functional components, for me, are the closest manifestation of Guillermo Rauch's concept of Pure UI.
Take for example, a video player component:
// Video.jsx
export default ({ src }) => <video src={src} />
Lovely stuff.
Intuitiveness
This was a pleasant surprise in Vue. If I wasn't sure how to do something, I found that if I tried to implement it as I saw fit (ie. guessed), 9 times out of 10...that's exactly how it was supposed to work. I find Vue incredibly intuitive. This goes hand in hand with their excellent documentation.
No setState
The ceremony and fluff that surrounds React's setState
drives me nuts. Also the fact that state updates are asynchronous. Not DOM updates (that I can understand), but updates to the state object itself, which seems 1) counter-intuitive and 2) doesn't feel obvious that that's the case. Add these two things together and you can have some painful debugging experiences.
// Counter.jsx
this.setState({
counter: ++this.state.counter
})
// Counter.vue
++this.counter
Pug
This is going to be an acquired taste, but honestly, the brevity of Pug has won me over. HTML (JSX) is a hecking pain to write. I know Emmet exists for autocomplete...
// Nav-bar.jsx
render() {
return (
<div className="nav-bar">
<div className="flex">
<div
className="logo"
loading={this.state.tweetsLoading}
onClick={this.scrollToTop}
/>
{(!!user) && <ThemeSwitcher />}
{(!!user) && <User />}
</div>
</div>
)
}
// Nav-bar.vue
<template lang="pug">
.nav-bar
.flex
.logo(v-loading="tweetsLoading", @click="scrollToTop")
theme-switcher(v-if="user")
user(v-if="user")
</template>
You get the picture.
Scoped CSS
Going back to React styling after working in Vue's scoped CSS world was jarring.
Pop this at the bottom of your Vue component:
<style lang="sass" scoped>
p
color: red
</style>
And only the <p />
tags inside your component are styled red, thanks to the scoped
attribute.
From the Vue docs:
One important thing to note is that separation of concerns is not equal to separation of file types.
Having your CSS right there next to your Pug (/HTML/JSX) is great. Switching to a different file to style something seems really disconnected now.
Implicit props
A really small thing, but in Vue, the props are automatically added to the instance. So you don't have this.state.foo
and this.props.bar
, you just have this.foo
and this.bar
. Brevity.
Ecosystem
I would say that React's ecosystem is only marginally better, it just feels like more people are using it. But to be honest, I can't really fault Vue's ecosystem at all. I just think React's is slightly more vast and lively. Everything I searched for with Vue, I found. Every plugin I wanted to install, it was there.
State management
React has Redux, Vue has Vuex. I like both. Both are good. That's all she wrote.
If all you have is a hammer, everything looks like a nail
Inevitably, React and Vue are just tools, they're not the singular solution. It's going to come down to personal preference. For me, neither of them stand out enough to convince me never to use the other. If you try really hard, most things can become your hammer.