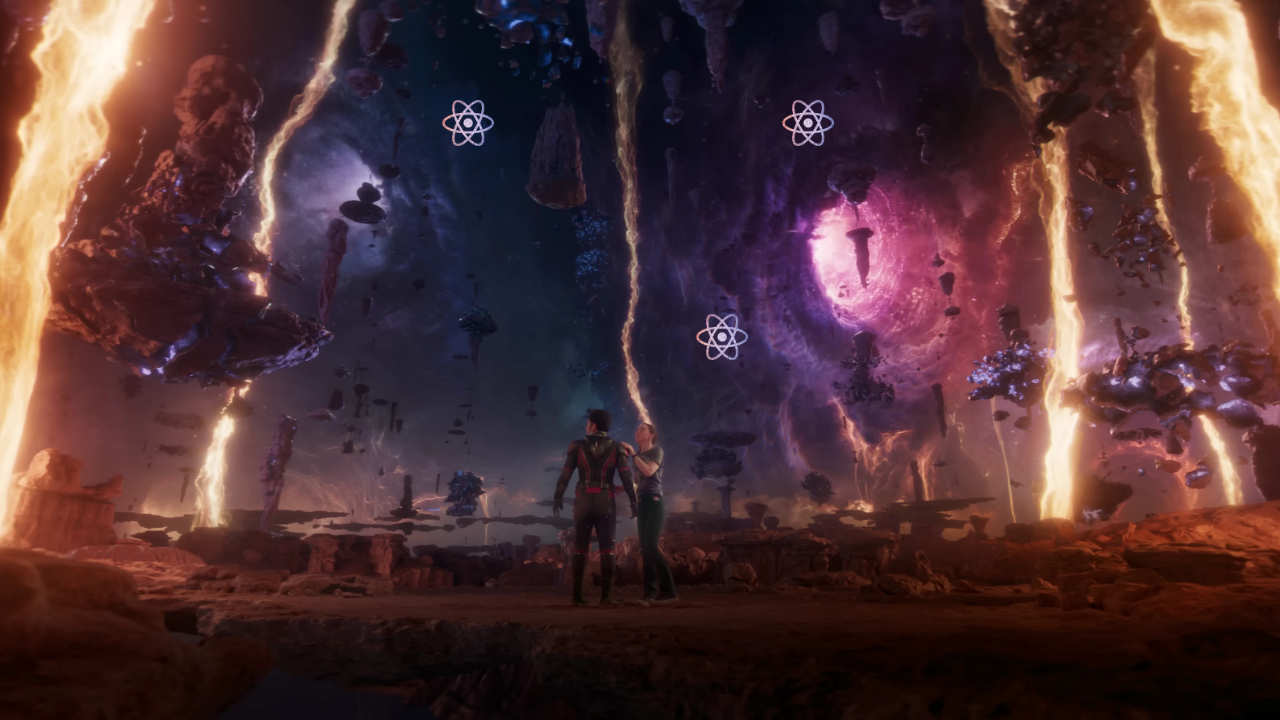
"Alright, five years ago, right before Thanos, I was in a place called the Quantum Realm. The Quantum Realm is like its own microscopic universe" - Scott Lang, Avengers: Endgame
Every line of code we write is an act of creation—a way of collapsing the infinite potential of an idea into something real. Yet, we rarely stop to consider: what if our UI components themselves could exist in a state of quantum potential? What if the principles of the quantum realm—superposition, entanglement, and collapse—could inspire how we design and build interfaces?
This is not a post about buzzwords (although, granted, it's rife with them). It's about rethinking the way we approach interface development. By applying quantum mechanics as a lens for UI design, we can unlock new ways to think about state, interaction, and composition. And perhaps—just perhaps—we can glimpse the future of what user interfaces might become.
Superposition: Designing for Potential, Not Certainty
In the quantum world, superposition describes a system existing in all possible states simultaneously until observed. In development, we often approach UI components as static entities: a button is either hovered or not, clicked or not, active or not. But what if we thought of them as systems of potential—existing in multiple states at once until the user's interaction resolves them?
Let's imagine a quantum-inspired button:
// MultiStateButton.tsx
import React, { useState } from "react";
type ButtonState = "default" | "hovered" | "clicked" | "disabled";
export const MultiStateButton: React.FC = () => {
const [state, setState] = useState<ButtonState>("default");
const handleMouseEnter = () => state === "default" && setState("hovered");
const handleMouseLeave = () => state === "hovered" && setState("default");
const handleClick = () =>
setState((prev) => (prev === "disabled" ? prev : "clicked"));
return (
<button
className={`multi-state-button ${state}`}
onMouseEnter={handleMouseEnter}
onMouseLeave={handleMouseLeave}
onClick={handleClick}
disabled={state === "disabled"}
>
{state === "clicked" ? "Clicked!" : "Hover or Click Me"}
</button>
);
};
This button doesn't assume its role—it waits for interaction to decide its state. The user is the observer who collapses the possibilities into a rendered interface. While this might feel contrived, it challenges us to consider: What if all components were designed to reflect dynamic, contextual possibilities instead of fixed states?
For example: A product card could display multiple prices (discounted, full price, subscription) simultaneously—resolved only when the user's context (location, preferences, or membership) becomes known. Superposition invites us to design for ambiguity, to embrace potential over certainty.
Entanglement: UI Components Beyond Isolation
In the quantum realm, entanglement binds particles so deeply that a change to one immediately affects the other, no matter the distance. In UI development, we often view components as modular and isolated. But real-world interactions are rarely that clean. How can we embrace a model where components are deeply and intrinsically connected?
Consider this: a collaborative text editor. An input field on one user's screen is entangled with another user's display. Any change ripples through the system instantaneously, not constricted by time or space or location:
// quantumState.tsx
import React, { createContext, useContext, useState } from "react";
type QuantumState = {
value: string;
setValue: (newValue: string) => void;
};
const QuantumContext = createContext<QuantumState | null>(null);
export const QuantumProvider: React.FC<{ children: React.ReactNode }> = ({
children,
}) => {
const [value, setValue] = useState("Initial Value");
return (
<QuantumContext.Provider value={{ value, setValue }}>
{children}
</QuantumContext.Provider>
);
};
export const useQuantumState = () => {
const context = useContext(QuantumContext);
if (!context)
throw new Error("useQuantumState must be used within QuantumProvider");
return context;
};
Now, two components—EntangledInput
and EntangledDisplay
—can share this state:
// EntangledInput.tsx
import React from "react";
import { useQuantumState } from "./quantumState";
export const EntangledInput: React.FC = () => {
const { value, setValue } = useQuantumState();
return (
<input
value={value}
onChange={(e) => setValue(e.target.value)}
placeholder="Type here, watch the magic"
/>
);
};
// EntangledDisplay.tsx
import React from "react";
import { useQuantumState } from "./quantumState";
export const EntangledDisplay: React.FC = () => {
const { value } = useQuantumState();
return <p>Entangled Value: {value}</p>;
};
This isn't just state-sharing—it's a philosophy. Entangled components move us away from "atomic design" to something more organic, more relational. Think of a global notification system: a user dismissing a banner updates not just their view, but every connected context where that banner appears. The connection becomes the system's lifeblood, agnostic to recipient or sender.
Quantum Collapse: The Power of Interaction
In quantum mechanics, collapse occurs when a system's infinite possibilities reduce to a single, observable state. For interfaces, this mirrors how interactions—clicks, taps, scrolls—determine what the user experiences next. But what if we stopped thinking of "conditional rendering" as a utility and started treating it as an art, or....a science?
Take a personalized onboarding wizard:
// OnboardingCard.tsx
import React, { useState } from "react";
export const OnboardingCard: React.FC = () => {
const [step, setStep] = useState(0);
const handleNext = () => setStep((prev) => prev + 1);
return (
<div className="onboarding-card">
{step === 0 && <p>Welcome! Let's get started.</p>}
{step === 1 && <p>Tell us more about yourself.</p>}
{step === 2 && <p>You're all set! Enjoy the journey.</p>}
<button onClick={handleNext}>{step < 2 ? "Next" : "Finish"}</button>
</div>
);
};
Here, each interaction collapses the UI into a new state. But what if this collapse wasn't linear? What if the UI responded probabilistically, surfacing the most relevant next step based on incomplete data? That's where machine learning meets quantum-inspired design, and the possibilities explode.
Turning Theory into Introspection
This isn't just a thought experiment. By applying quantum principles to React, we can reimagine how we approach state, interaction, and composition:
- Superposition asks us to design for ambiguity and adaptability.
- Entanglement challenges us to think relationally, connecting components at a deeper level.
- Collapse reminds us that interaction isn't just a transition—it's a moment of transformation.
What's more exciting is what we haven't explored. How might quantum-first interfaces look in the age of quantum computing? Could UIs leverage probabilistic algorithms to predict user intent? Could they dynamically adapt based on entangled user profiles across systems? These are the questions worth asking—not just for React developers, but for anyone rethinking what a user interface can be.
Ultimately, quantum UI design isn't about the technology—it's about the mindset. It's an invitation to create systems that are dynamic, responsive, and deeply human. And the best part? This isn't theoretical. It's happening now, in the decisions you make every day.
A Quantum leaping off point
Below is a conceptual React library designed to bring quantum-inspired principles into UI development. It introduces abstractions like Superposition
, Entanglement
, and Collapse
to help developers create more dynamic, adaptive, and interconnected components.
Superposition
allows components to represent multiple states simultaneously, resolving into one based on runtime conditions. This abstraction is perfect for dynamic content that depends on user context or probabilistic logic.Entanglement
enables seamless state sharing between components, creating deeply interconnected systems. This is ideal for collaborative features or global state synchronization.Collapse
treats conditional rendering as a transformative interaction, enabling UIs to elegantly evolve based on user input.
By applying these abstractions, we can simplify complex state transitions, enhances the fluidity of shared data, and empowers developers to design UIs that feel alive and responsive to user intent.
// src/quantum/Superposition.tsx
import React, { ReactNode, useState, useEffect } from "react";
/**
* Superposition: A wrapper that accepts multiple states and resolves to one based on a condition.
*/
type SuperpositionProps<T> = {
states: T[];
resolver: (states: T[]) => T;
children: (state: T) => ReactNode;
};
export function Superposition<T>({
states,
resolver,
children,
}: SuperpositionProps<T>) {
const [resolvedState, setResolvedState] = useState<T>(states[0]);
useEffect(() => {
const result = resolver(states);
setResolvedState(result);
}, [states, resolver]);
return <>{children(resolvedState)}</>;
}
// src/quantum/Entanglement.tsx
import React, { createContext, useContext, useState, ReactNode } from "react";
/**
* EntanglementContext for shared state
*/
type EntanglementContextValue<T> = {
value: T;
setValue: (newValue: T) => void;
};
const createEntanglement = <T,>(defaultValue: T) => {
const EntanglementContext = createContext<EntanglementContextValue<T> | null>(
null
);
const EntanglementProvider: React.FC<{ children: ReactNode }> = ({
children,
}) => {
const [value, setValue] = useState(defaultValue);
return (
<EntanglementContext.Provider value={{ value, setValue }}>
{children}
</EntanglementContext.Provider>
);
};
const useEntangledState = (): EntanglementContextValue<T> => {
const context = useContext(EntanglementContext);
if (!context) {
throw new Error(
"useEntangledState must be used within an EntanglementProvider"
);
}
return context;
};
return { EntanglementProvider, useEntangledState };
};
export const Entanglement = createEntanglement;
// src/quantum/Collapse.tsx
import React, { ReactNode, useState } from "react";
/**
* Collapse: Renders a component based on user interaction or conditions.
*/
type CollapseProps = {
trigger: ReactNode;
children: ReactNode;
};
export const Collapse: React.FC<CollapseProps> = ({ trigger, children }) => {
const [isOpen, setIsOpen] = useState(false);
return (
<div>
<div onClick={() => setIsOpen((prev) => !prev)}>{trigger}</div>
{isOpen && <div>{children}</div>}
</div>
);
};
// Example usage in App.tsx
import React from "react";
import { Superposition } from "./quantum/Superposition";
import { Collapse } from "./quantum/Collapse";
import { Entanglement } from "./quantum/Entanglement";
const { EntanglementProvider, useEntangledState } =
Entanglement<string>("Default Value");
const InputComponent: React.FC = () => {
const { value, setValue } = useEntangledState();
return <input value={value} onChange={(e) => setValue(e.target.value)} />;
};
const DisplayComponent: React.FC = () => {
const { value } = useEntangledState();
return <p>Entangled Value: {value}</p>;
};
export const App: React.FC = () => (
<div>
<h1>Superposition Example</h1>
<Superposition
states={["Loading...", "Welcome!", "Goodbye!"]}
resolver={(states) => states[Math.floor(Math.random() * states.length)]}
>
{(state) => <h2>{state}</h2>}
</Superposition>
<h1>Entanglement Example</h1>
<EntanglementProvider>
<InputComponent />
<DisplayComponent />
</EntanglementProvider>
<h1>Collapse Example</h1>
<Collapse trigger={<button>Show More</button>}>
<p>This content is revealed when the collapse is triggered!</p>
</Collapse>
</div>
);
Quantum Leapt
Quantum mechanics has long been a source of wonder, mystery, and paradigm-shifting insights into the nature of reality. By borrowing its principles to inspire how we build interfaces, we unlock new ways to think about the systems we create. Superposition teaches us to embrace ambiguity and design for potential, entanglement reminds us of the interconnectedness of data and experience, and collapse challenges us to view interaction as transformational rather than transactional. As developers, we are not just building UIs—we are crafting experiences that shape how users interact with technology and the world. The real power of these quantum-inspired ideas isn't in the code itself, but in the mindset they cultivate: one that values adaptability, connection, and endless possibility. The question now isn't whether to embrace these concepts, but how far they can take us.